목표 및 문제정의
- do-with 프로젝트에서 shadcn UI 라이브러리를 사용해서 "가입 대기 스페이스"를 보여주기 위해 popover 컴포넌트를 활용했다.
- 아래는 반응형 디자인에서 각 화면 크기에서 "file" 이미지를 클릭했을 때 "가입 대기 스페이스" UI가 나타나는 모습이다. 모든 화면 크기에서 "참여중인 스페이스"의 너비와 동일한 너비가 적용되도록 디자인했다.
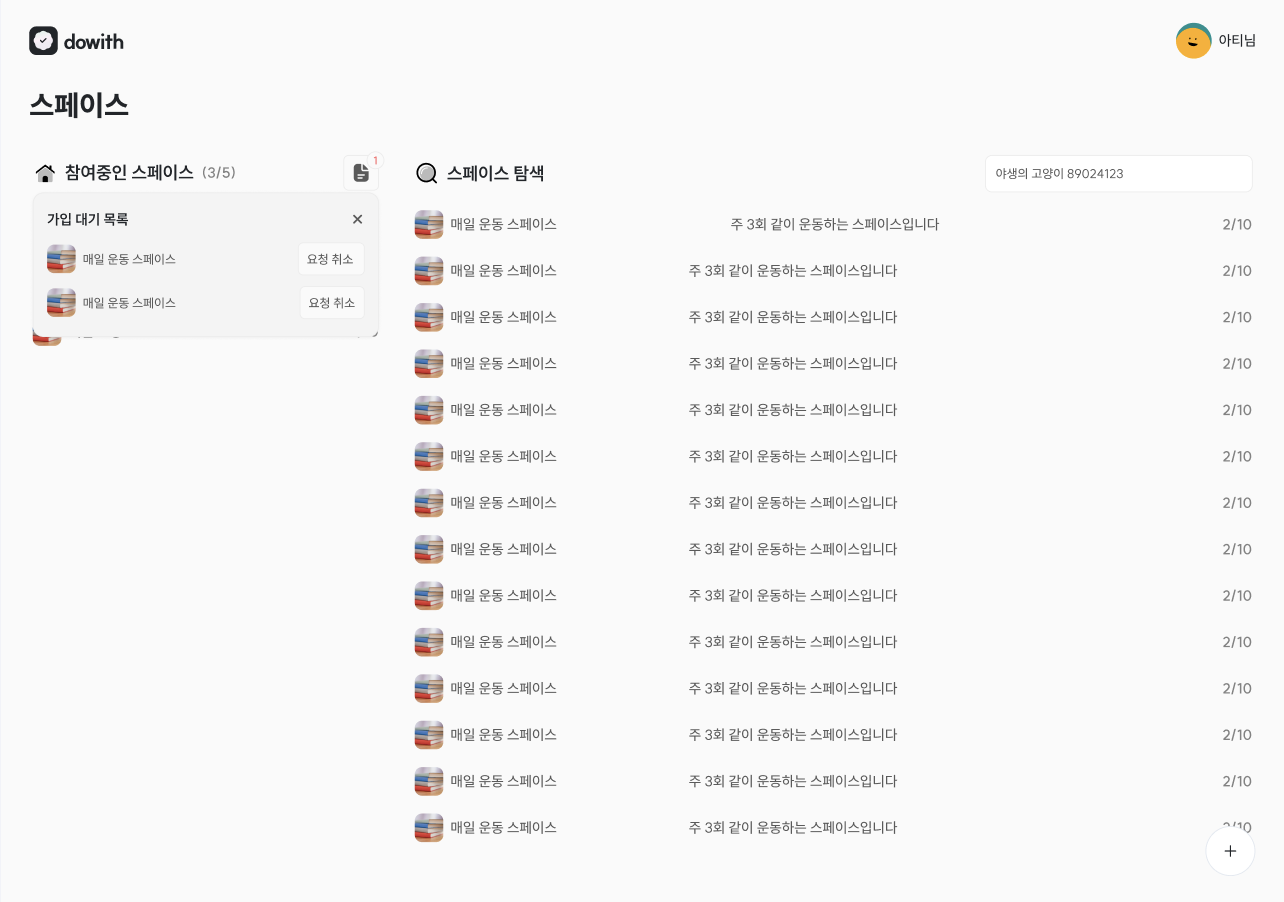
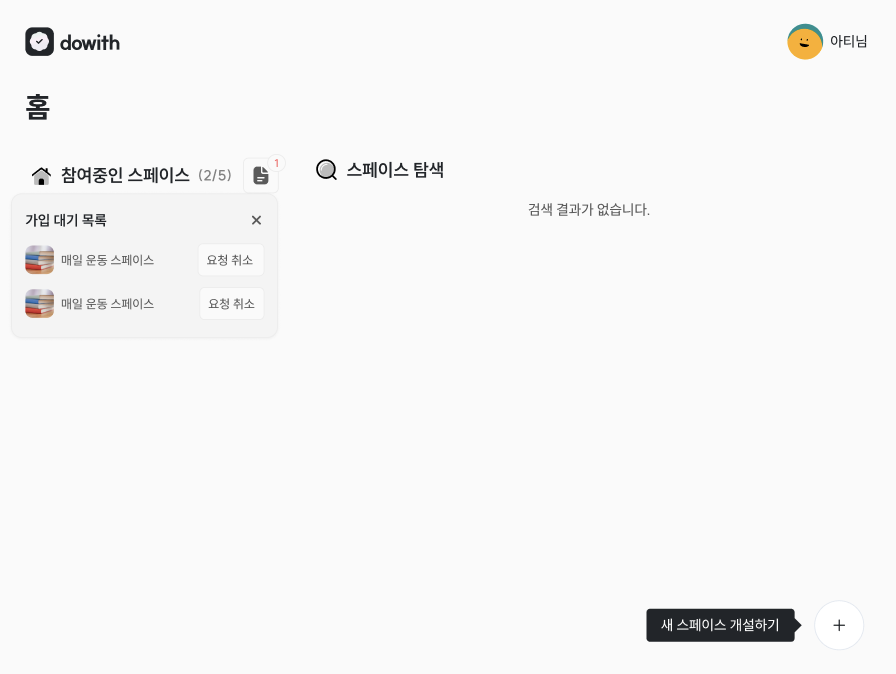
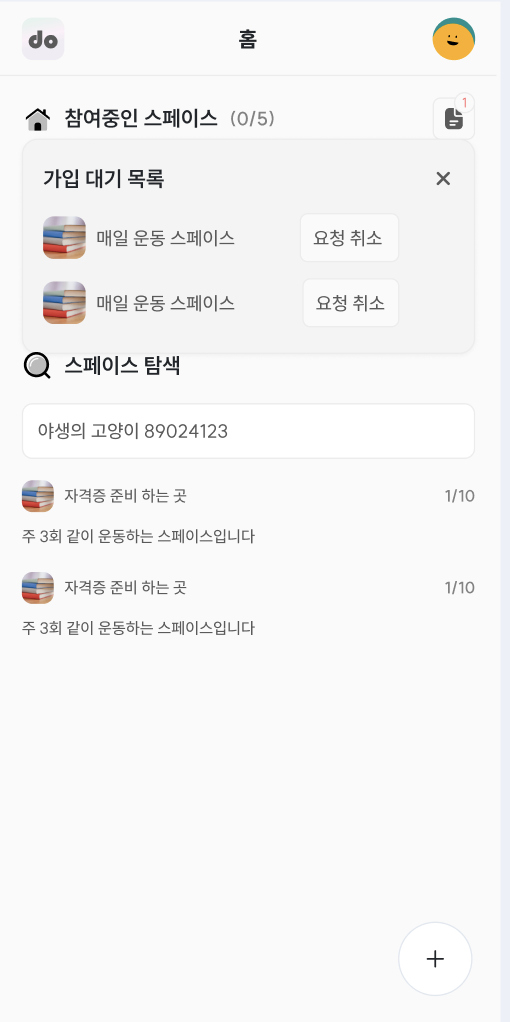
- 하지만 문제는 Popove 컴포넌트가 React portal을 사용해 렌더링되면서 발생했다. Portal은 DOM 트리의 계층을 무시하고 컴포넌트를 body의 직속 자식 요소로 렌더링하기 때문에, Popover가 "참여중인 스페이스"와 같은 DOM 트리 안에 위치하지 않게 되었다.
- 이로 인해 반응형 디자인에서 "참여중인 스페이스"의 너비가 변경되더라도, Popover의 너비를 "참여중인 스페이스"와 동일하게 동기화하기 어려운 문제가 있었다. Popover가 DOM 트리 외부에 렌더링되기 때문에 부모 요소의 width를 직접 참조할 수 없었기 때문이다.
해결방법
- 첫 번째 해결 방법으로, "참여중인 스페이스"의 너비를 prop으로 "가입 대기 스페이스" 컴포넌트에 전달하는 방법을 선택했다. 이를 위해 ResizeObserver를 사용해 "참여중인 스페이스" 컴포넌트를 관찰하고 변경된 너비를 state로 관리하였다.
- ResizeObserver란 DOM 요소의 크기(가로, 세로) 변화를 관찰할 수 있는 브라우저 API다. 특정 요소의 크기가 변할 때마다 실행할 콜백 함수를 등록해서 크기 변화에 따라 동작을 트리거할 수 있게 도와준다.
- 실제 코드를 보면 다음과 같다.
// 특정 DOM 요소의 너비를 추적하는 useElementWidth 훅
export const useElementWidth = <T extends HTMLElement>(isActive?: boolean) => {
// 관찰할 요소의 참조를 저장하는 ref
const elementRef = useRef<T>(null);
// 요소의 현재 너비를 상태로 관리
const [width, setWidth] = useState<number>(0);
useEffect(() => {
// ref가 초기화되지 않았으면 종료
if (!elementRef.current) return;
// 초기 너비 설정
setWidth(elementRef.current.clientWidth);
// ResizeObserver를 생성하여 요소 크기 변화를 관찰
const observer = new ResizeObserver((entries) => {
for (const entry of entries) {
if (entry.contentRect) {
// 크기 변화가 감지되면 상태 업데이트
// 디바운스 처리가 필요한 경우, 적절한 방법을 추가할 수 있음
setWidth(entry.contentRect.width);
}
}
});
// 관찰할 요소 등록
observer.observe(elementRef.current);
// 컴포넌트 언마운트 시 관찰 중단
return () => {
observer.disconnect();
};
}, [isActive]); // isActive 상태가 변경될 때만 useEffect 실행
// ref와 현재 너비를 반환
return { elementRef, width };
};
// HomePage.tsx
// useElementWidth 훅을 활용하여 참여중인 스페이스의 너비 확인
const { elementRef: joinedSpaceSectionRef, width: joinedSpaceSectionWidth } =
useElementWidth<HTMLDivElement>(isCheckingAuth);
...
return(
...
<ContentWrapper>
{/* 참여중인 스페이스 */}
<JoinedSpaceSection ref={joinedSpaceSectionRef}>
<JoinedSpaceSectionHeader>
<TitleAndWaitButtonWrapper>
<JoinedSpaceTitleWrapper>
<HomeIcon className="size-7 md:size-6" />
<JoinedSpaceTitle>참여중인 스페이스</JoinedSpaceTitle>
<JoinedSpaceCount>
{joinedSpaceList.length} / {MAX_SPACES_PER_USER}
</JoinedSpaceCount>
</JoinedSpaceTitleWrapper>
{/* 가입 대기 스페이스 */}
<WaitingSpaceList containerWidth={joinedSpaceSectionWidth} />
</TitleAndWaitButtonWrapper>
{joinedSpaceList.length > 0 && (
<JoinedSpaceDescription>
스페이스를 클릭하면 해당 스페이스 홈으로 이동합니다.
</JoinedSpaceDescription>
)}
</JoinedSpaceSectionHeader>
<JoinedSpaceList spaceData={joinedSpaceList} />
</JoinedSpaceSection>
<SearchedSpaceSection>
<SearchedSpaceList />
</SearchedSpaceSection>
</ContentWrapper>
...)
}
// 가입 대기 스페이스
export const WaitingSpaceList: React.FC<{ containerWidth?: number }> = ({
containerWidth,
}) => {
const [isPopoverOpen, setIsPopoverOpen] = useState(false);
const handleDeleteWaitingSpace = (requestId: number) => {
..
};
return (
<Popover open={isPopoverOpen} onOpenChange={setIsPopoverOpen}>
<PopoverTrigger asChild>
<WaitingSpaceDropdownButton>
<FileIcon className="size-7 md:size-6" />
<WaitingSpaceCountWrapper>
<WaitingSpaceCount>{spaceData.length}</WaitingSpaceCount>
</WaitingSpaceCountWrapper>
</WaitingSpaceDropdownButton>
</PopoverTrigger>
{/* prop으로 전달받은 참여중인 스페이스 너비로 스타일 처리 */}
<StyledPopoverContent
style={{ width: `${containerWidth}px` }}
align="end"
sideOffset={0}
>
<WaitingSpaceWrapper>
<WaitingSpaceListHeader>
<WaitingSpaceListTitle>가입 대기 목록</WaitingSpaceListTitle>
<CloseIcon
className="size-5 md:size-4 cursor-pointer"
onClick={() => {
setIsPopoverOpen(false);
}}
/>
</WaitingSpaceListHeader>
<SpaceList>
...
</SpaceList>
</WaitingSpaceWrapper>
</StyledPopoverContent>
</Popover>
);
};
- 특정 요소의 width 변화를 감지하고 이를 반환하는 useElementWidth 커스텀 훅을 작성했다.
- 이를 사용해 "참여중인 스페이스"의 요소를 관찰하고, 해당 요소의 너비를 prop으로 "가입 대기 스페이스" 컴포넌트에 전달하여 너비를 기준으로 스타일링 했다.
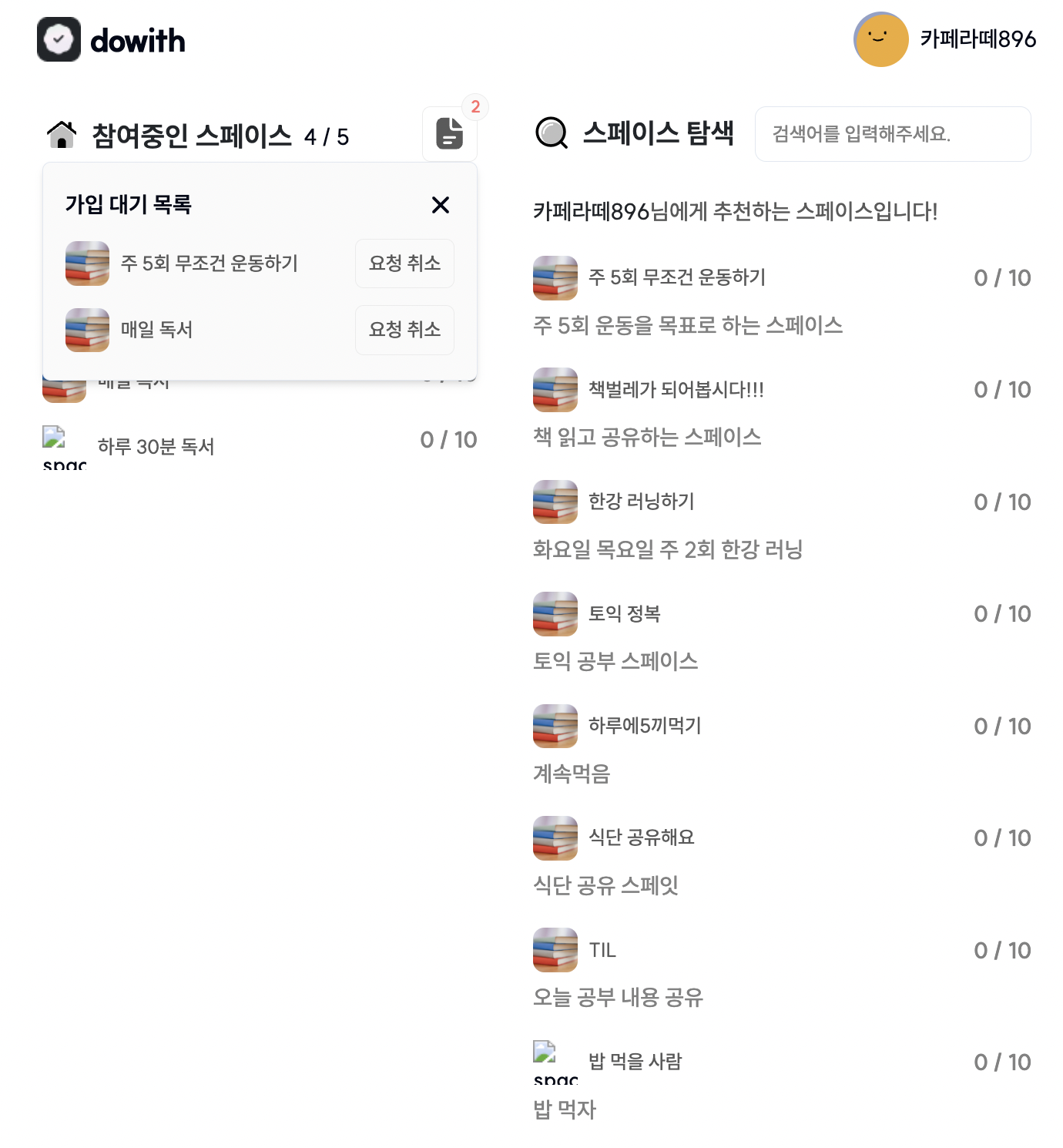
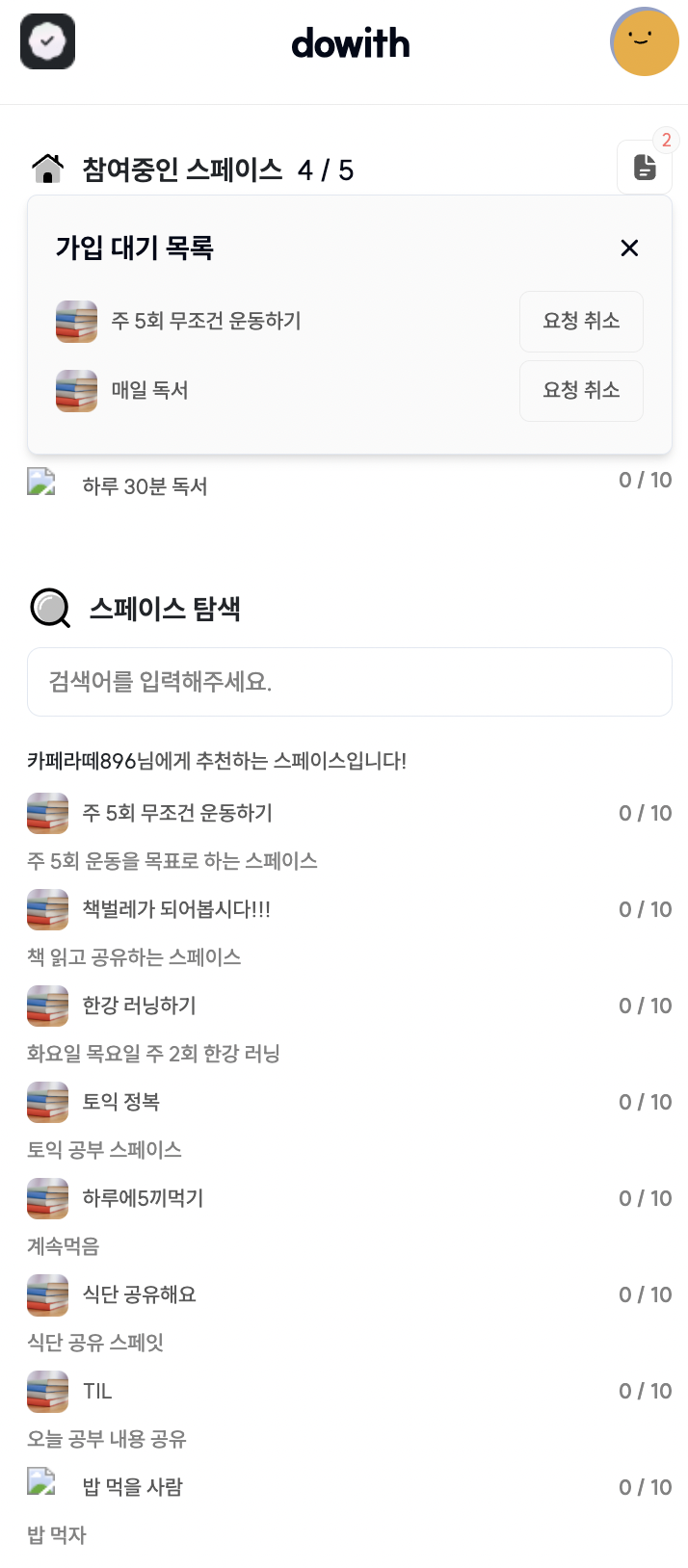
- 위 이미지는 코드가 적용된 실제 화면으로, "가입 대기 스페이스"의 너비는 화면 크기에 따라 달라지는 "참여중인 스페이스"의 너비에 맞춰 변하는 걸 볼 수 있다.
- 하지만, 작성된 코드의 단점이 있다!
- 화면 크기가 변할 때, 더 구체적으로는 "참여중인 스페이스"의 너비가 변경될 때마다 useElementWidth에서 관리하는 상태(width)가 지속적으로 업데이트되면서 불필요한 리렌더링이 과도하게 발생했다.
- 이를 해결하기 위해 디바운스를 적용하여, 일정 시간 내의 마지막 너비 변화만을 반영하도록 하여 리렌더링 횟수를 줄였다.
// useElementWidth.ts
import { useState, useEffect, useRef } from 'react';
// 디바운스 처리 함수
// 특정 시간(`timeout`) 내에 여러 번 호출된 값 중 가장 마지막 값만 반영
type DebounceSetWidthCallback<T> = (value: T) => void;
const debounceSetWidth = <T>(
callback: DebounceSetWidthCallback<T>, // 실행할 콜백 함수
timeout = 300 // 디바운스 간격(기본값 300ms)
) => {
let timer: ReturnType<typeof setTimeout> | null = null; // 타이머를 저장할 변수
return (value: T) => {
if (timer) clearTimeout(timer); // 이전 타이머 제거
timer = setTimeout(() => {
callback(value); // 일정 시간 후 콜백 실행
}, timeout);
};
};
// 특정 DOM 요소의 너비를 추적하는 커스텀 훅
export const useElementWidth = <T extends HTMLElement>(isActive?: boolean) => {
const elementRef = useRef<T>(null); // 관찰할 DOM 요소의 ref
const [width, setWidth] = useState<number>(0); // 요소의 현재 너비를 관리하는 상태
useEffect(() => {
// elementRef가 초기화되지 않았다면 아무 작업도 하지 않음
if (!elementRef.current) return;
// 디바운스된 setWidth 함수 생성
const debouncedSetWidth = debounceSetWidth(setWidth, 500);
// 초기 너비 설정
setWidth(elementRef.current.clientWidth);
// ResizeObserver를 생성하여 요소 크기 변화를 감지
const observer = new ResizeObserver((entries) => {
for (const entry of entries) {
if (entry.contentRect) {
// 크기 변화를 디바운스 처리하여 상태 업데이트
debouncedSetWidth(entry.contentRect.width);
}
}
});
// 관찰할 요소 등록
observer.observe(elementRef.current);
// 컴포넌트 언마운트 시 관찰 중단
return () => {
observer.disconnect();
};
}, [isActive]); // isActive 값이 변경될 때마다 useEffect 실행
// ref와 현재 너비를 반환
return { elementRef, width };
};
- 하지만 이 접근법에도 한계가 있다.
- Popover가 열려 있어 "가입 대기 스페이스"가 화면에 렌더링된 상태에서 사용자가 화면 크기를 조정할 경우, 디바운스 처리로 인해 너비 변화가 즉각적으로 반영되지 않는 문제가 발생한다.
- 즉, "참여중인 스페이스"의 너비를 관리할 때, state로 관리하면 불필요한 리렌더링이 발생하고, 디바운스를 적용하면 너비 변화가 즉각적으로 반영되지 않는 문제가 있다. 따라서, "참여중인 스페이스"의 너비를 state로 관리하는 대신, CSS를 활용하여 해결해야 한다고 판단했다.
- 방법은 "참여중인 스페이스"의 너비를 CSS 변수로 정의하고, "가입 대기 스페이스"의 너비에 해당 CSS 변수를 적용하는 것인데, 이전의 방법과 비슷하게 ResizeObserver를 사용했다.
최종 적용 코드
// useResizeCssVariable.tsx
// 특정 DOM 요소의 크기를 관찰하여 CSS 변수에 동적으로 업데이트하는 커스텀 훅
import { useRef, useEffect } from 'react';
export const useResizeCssVariable = <T extends HTMLElement>(
variableName: string, // 동적으로 업데이트할 CSS 변수 이름
offset: number = 0, // 크기에 더하거나 빼는 오프셋 값 (기본값: 0)
dependency?: boolean // 의존성 배열로 사용할 값 (기본값: 없음)
) => {
const elementRef = useRef<T>(null); // 대상 DOM 요소를 참조하기 위한 useRef
useEffect(() => {
const element = elementRef.current; // 참조된 DOM 요소 가져오기
if (!element) return; // 요소가 없으면 종료
const updateCssVariable = () => {
// 요소의 현재 너비에 오프셋을 더한 값을 CSS 변수로 설정
const computedWidth = `${element.offsetWidth + offset}px`;
document.documentElement.style.setProperty(variableName, computedWidth);
};
updateCssVariable(); // 초기 CSS 변수 설정
const resizeObserver = new ResizeObserver((entries) => {
// 요소 크기 변화를 관찰
for (const entry of entries) {
if (entry.contentRect) {
// 요소의 크기가 변하면 CSS 변수 업데이트
updateCssVariable();
}
}
});
resizeObserver.observe(element); // 요소를 ResizeObserver로 관찰
return () => {
resizeObserver.disconnect(); // 컴포넌트 언마운트 시 Observer 해제
document.documentElement.style.removeProperty(variableName); // CSS 변수 제거
};
}, [variableName, offset, dependency]); // 의존성 배열: CSS 변수 이름, 오프셋, 외부 의존성
return { elementRef }; // 요소를 참조할 수 있도록 반환
};
// HomePage.tsx
export const HomePage = () => {
// 로그인 여부를 확인하는 훅
const isCheckingAuth = useAuthCheckAndRedirectLogin();
const { elementRef: joinedSpaceSectionRef } =
useResizeCssVariable<HTMLDivElement>(
'--joined-space-section-width',
-30,
isCheckingAuth
);
// ...
return (
...
// 참여중인 스페이스에 ref 지정
<JoinedSpaceSection ref={joinedSpaceSectionRef}>
<JoinedSpaceSectionHeader>
<TitleAndWaitButtonWrapper>
<JoinedSpaceTitleWrapper>
<HomeIcon className="size-7 md:size-6" />
<JoinedSpaceTitle>참여중인 스페이스</JoinedSpaceTitle>
<JoinedSpaceCount>
{joinedSpaceList.length} / {MAX_SPACES_PER_USER}
</JoinedSpaceCount>
</JoinedSpaceTitleWrapper>
<WaitingSpaceList />
</TitleAndWaitButtonWrapper>
{joinedSpaceList.length > 0 && (
<JoinedSpaceDescription>
스페이스를 클릭하면 해당 스페이스 홈으로 이동합니다.
</JoinedSpaceDescription>
)}
</JoinedSpaceSectionHeader>
<JoinedSpaceList spaceData={joinedSpaceList} />
</JoinedSpaceSection>
...
)}
// WaitingSpaceList.tsx
export const WaitingSpaceList = () => {
...
return (
<Popover>
...
<StyledPopoverContent align="end" sideOffset={0}>
<WaitingSpaceWrapper>
<WaitingSpaceListHeader>
<WaitingSpaceListTitle>가입 대기 목록</WaitingSpaceListTitle>
<CloseIcon
className="size-5 md:size-4 cursor-pointer"
onClick={() => {
setIsPopoverOpen(false);
}}
/>
</WaitingSpaceListHeader>
...
</Popover>
);
};
// css 변수 사용
const StyledPopoverContent = styled(PopoverContent)`
${tw`bg-white px-4 py-5 [width:var(--joined-space-section-width)]`}
`;
적용 화면
후기
- 역시 스타일을 적용하는 것은 항상 쉽지 않다는 것을 또 한번 느꼈다.🫠
학습 단계로 잘못된 정보가 있을 수 있습니다. 잘못된 부분에 대해 알려주시면 정정하도록 하겠습니다
참고 : https://velog.io/@leeji/ResizeObserver
'프로젝트 > dowith' 카테고리의 다른 글
Zustand를 활용한 모달 상태 관리 (0) | 2024.11.02 |
---|---|
Axios interceptors를 활용하여 액세스 & 리프레시 토큰 자동 갱신 구현하기 (0) | 2024.09.07 |
twin.macro 도입 및 Tailwind CSS + styled-components (0) | 2024.09.07 |